Introduction
Markdown is a lightweight and easy-to-use format for writing formatted text using plain-text editors such as Notepad and nano
. The format is widely used in documentation, blogs, and platforms like GitHub for README files. The markdown format makes writing on the web simpler and more intuitive. You can use simple tags like asterisks (*) for emphasis or hashtags (#) for headings to create rich text elements such as bold, italics, lists, links, and tables without complex HTML tags.
This guide shows you how to use the Python Markdown library to render Markdown content as HTML.
Prerequisites
Before you start:
- Deploy a Digital Ocean VPS server running Ubuntu, Debian, Rocky Linux, AlmaLinux, CentOS Stream, Arch Linux, or Fedora.
- Create a non-root sudo user. For instructions, see the guide below:
- Install Python. Check the instructions in the following guide:
Install the Python Markdown Library
Before using the Markdown , use pip
, a package manager for Python to install the Markdown library. You'll install the library in a new virtual environment.
-
Create a directory for your project.
CONSOLE$ mkdir project
-
Switch to the new directory.
CONSOLE$ cd project
-
Install the
python3-venv
package, which provides tools for creating and managing virtual environments in Python.-
Debian/Ubuntu
CONSOLE$ sudo apt install python3-venv
-
Rocky Linux/Cent OS.
CONSOLE$ sudo dnf install python3 python3-virtualenv
-
Fedora
CONSOLE$ sudo dnf install python3-virtualenv
-
Arch Linux
CONSOLE$ sudo pacman -S python
-
-
Create a virtual environment.
CONSOLE$ python3 -m venv myenv
-
Activate the virtual environment.
CONSOLE$ source myenv/bin/activate
-
Install the
markdown
library.CONSOLE$ pip install markdown
Output:
Installing collected packages: markdown Successfully installed markdown-3.7
-
Ensure the library runs on your system.
CONSOLE$ pip show markdown
Output.
Name: Markdown Version: 3.7 Summary: Python implementation of John Gruber's Markdown. ...
Render Markdown from a String
To understand how the Python Markdown library works, you'll first render markdown content from a Python string. Use the Python triple quotes string literals to create a sample Markdown content and pass the plain text to the Markdown library to format it to HTML by following the steps below.
-
Create a new
markdown_script.py
file using thenano
text editor.CONSOLE$ nano markdown_script.py
-
Copy and paste the following code into the
markdown_script.py
file.Pythonimport markdown # Markdown text md_text = """ # Hello, Markdown! This is a **bold** word and this is an *italicized* word. """ # Convert Markdown to HTML html = markdown.markdown(md_text) # Display the HTML output print(html)
-
Save the file by pressing Ctrl + X, then Y and Enter.
-
Run the file using Python 3.
CONSOLE$ python3 markdown_script.py
You should see the HTML output of the Markdown string in the terminal.
HTML<h1>Hello, Markdown!</h1> <p>This is a <strong>bold</strong> word and this is an <em>italicized</em> word.</p>
Render Markdown from a File
When working with Python Markdown in a production environment, most of your content will usually come from files or a database. In this step, you'll create a sample .md
file and open the file using Python to render the content using the Markdown library.
-
Create a new
example.md
file.CONSOLE$ nano example.md
-
Add the following Markdown content to the
example.md
file. The sample below contains most common Markdown tags.Markdown# Welcome to Markdown Markdown is a lightweight markup language that uses plain text formatting. ## Subheading Example *This text is italicized* and **this text is bold**. * Here is a bullet point. * And another one. 1. Here is a numbered list item. 2. And another one. ### Blockquotes and Code Blocks > This is a blockquote. Use it to highlight important content. Here is a code block: print("Hello, Markdown!") ### Links and Images [Visit Python's website](https://www.python.org) 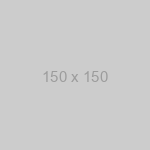 ### Tables | Column 1 | Column 2 | Column 3 | |----------|----------|----------| | Data 1 | Data 2 | Data 3 | | Data 4 | Data 5 | Data 6 | ### Lorem Ipsum Text Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
-
Save and close the file.
-
Remove the old
markdown_script.py
Python file.CONSOLE$ rm markdown_script.py
-
Recreate and open the
markdown_script.py
file usingnano
.CONSOLE$ nano markdown_script.py
-
Add the following Python code to the
markdown_script.py
file.Pythonimport markdown # Open and read the Markdown file with open('example.md', 'r') as md_file: md_text = md_file.read() # Convert Markdown to HTML html = markdown.markdown(md_text) # Display the HTML output print(html)
-
Save and close the file.
-
Run the file using Python 3.
CONSOLE$ python3 markdown_script.py
You should now see the HTML output of the Markdown file in the terminal.
HTML<h1>Welcome to Markdown</h1> <p>Markdown is a lightweight markup language that uses plain text formatting.</p> <h2>Subheading Example</h2> <p><em>This text is italicized</em> and <strong>this text is bold</strong>.</p> <ul> <li>Here is a bullet point.</li> <li> <p>And another one.</p> </li> <li> <p>Here is a numbered list item.</p> </li> <li>And another one.</li> </ul> <h3>Blockquotes and Code Blocks</h3> <blockquote> <p>This is a blockquote. Use it to highlight important content.</p> </blockquote> <p>Here is a code block:</p> <pre><code>print("Hello, Markdown!") </code></pre> <h3>Links and Images</h3> <p><a href="https://www.python.org">Visit Python's website</a></p> <p><img alt="Sample Image" src="https://via.placeholder.com/150" /></p> <h3>Tables</h3> <p>| Column 1 | Column 2 | Column 3 | |----------|----------|----------| | Data 1 | Data 2 | Data 3 | | Data 4 | Data 5 | Data 6 |</p> <h3>Lorem Ipsum Text</h3> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</p>
Render Python Markdown to an HTML Template
If you have several Markdown files or records coming from a database, the best way to render the Markdown in Python is to integrate the files into an HTML template and serve the content using Python built-in HTTP server. Follow the steps below.
-
Create a new
serve_markdown_script.py
fileCONSOLE$ nano serve_markdown_script.py
-
Add the following Python code to the
serve_markdown_script.py
file.Pythonimport markdown from http.server import HTTPServer, SimpleHTTPRequestHandler import os # Read Markdown file and convert to HTML with open('example.md', 'r') as md_file: md_text = md_file.read() html_content = markdown.markdown(md_text) # HTML Template html_template = f""" <!DOCTYPE html> <html> <head> <title>Markdown Rendered</title> </head> <body> {html_content} </body> </html> """ # Write the HTML content to a file with open('index.html', 'w') as html_file: html_file.write(html_template) # Serve the file on localhost:8090 class CustomHandler(SimpleHTTPRequestHandler): def do_GET(self): self.path = 'index.html' return SimpleHTTPRequestHandler.do_GET(self) httpd = HTTPServer(('', 8090), CustomHandler) print("Serving on port 8090...") httpd.serve_forever()
-
Save and close the file.
-
Open port
8090
depending on your Linux distribution:-
Ubuntu/Debian.
CONSOLE$ sudo ufw allow 8090 $ sudo ufw reload
-
Rocky Linux/AlmaLinux/CentOS Stream.
CONSOLE$ sudo firewall-cmd --add-port=8090/tcp --permanent $ sudo firewall-cmd --reload
-
Arch Linux/Fedora.
CONSOLE$ sudo firewall-cmd --add-port=8090/tcp --permanent $ sudo systemctl restart firewalld
-
-
Run the file using Python 3.
CONSOLE$ python3 serve_markdown_script.py
Output.
Serving on port 8090...
-
Visit the following URL on your browser. Replace
192.168.0.1
with your server's public IP address.http://192.168.0.1:8090
The Python Markdown library should now render the content as HTML.
Conclusion
This guide explains how to install and use the Python Markdown library to render Markdown into HTML, handling content from strings, files, and templates. It also includes step-by-step instructions for creating Python files with nano
, running the files using python3
, and configuring port 8090
for different Linux distributions. With this knowledge, you can efficiently handle and render Markdown content in your Python applications.